I've seen a number of blog posts floating around today about GMail Desktop Notifications (here, here, here, here and here -- did I miss anyone?). I tried them out myself and they are very useful. Being a rich web applications developer I, of course, wanted to figure out how it works and how I could use it for my own apps. Here's a quick overview of what I found.
There is a proposed web notification standard over at the W3C that Google submitted earlier this month (you can find it on the W3C site) but from what I can tell, that draft isn't implemented in any browsers (including Google Chrome!). But you can get desktop notifications today without the need for any third-party browser extensions, if you're using Google Chrome. You can find the documentation over on the Chromium developer site. The rest of this post is a collection of quick examples I put together for using notifications:
Checking for permission
It is pretty easy to check to see if the user has allowed notifications for your website. The first example displays an alert depending on the permission level.
switch ( webkitNotifications.checkPermission() )
{
case 0: // PERMISSION_ALLOWED
alert( "Permission: allowed" );
break;
case 1: // PERMISSION_NOT_ALLOWED
alert( "Permission: not allowed" );
break;
case 2: // PERMISSION_DENIED
alert( "Permission: denied" );
break;
}
Try it: Check Permission
Unless you skipped ahead and tried the next bit of code, the answer is "No" my site doesn't have permission to display notifications.
Requesting Permission
So before we do anything we need to ask for permission.
This method will only work when responding to a user gesture. Which means you can call it as a direct response to an action taken by the user; but you can't call it (for example) on the response to an Ajax function. So if you wanted to call it when your Ajax call returns or when a timer fires, you're out of luck. You need to request permission before then.
webkitNotifications.requestPermission();
Try it: Request Permission
Creating and Showing Notification
Notably, showing a standard browser alert dialog when permission is denied is a terrible user experience. Don't do that! This is just for demo purposes.
if ( webkitNotifications.checkPermission() == 0 )
{
var iconImageUrl = "http://www.example.com/foo.png";
var title = "Hello World";
var subTitle = "This is a sample desktop notification."
var notification = webkitNotifications.createNotification( iconImageUrl, title, subTitle );
notification.show();
}
else
{
alert( "Please request permissions first." );
}
Try it: Show Notification
The new notification looks something like this (for those of you not using Chrome and want to see what I am talking about):
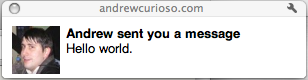
Creating and Showing an HTML Notification
You can also create a notification by passing in a URL.
if ( webkitNotifications.checkPermission() == 0 )
{
var url = "http://www.example.com/notification.html";
var notification = webkitNotifications.createHTMLNotification( url );
notification.show();
}
else
{
alert( "Please request permissions first." );
}
Try it: Show Notification
At first this seems like a terrible idea. It could be yet another way to have annoying advertisements pop up on a site. But it's not as bad as it sounds since the user has to explicitly allow the notifications and they can be turned off at any time.
One Final Example
Now, you probably don't want the notification to pop up and stay there forever. So, you can auto-hide it using a timer.
if ( webkitNotifications.checkPermission() == 0 )
{
var iconImageUrl = "http://www.example.com/foo.png";
var title = "Hello World";
var subTitle = "This is a sample desktop notification."
var notification = webkitNotifications.createNotification( iconImageUrl, title, subTitle );
notification.show();
setTimeout( function() { notification.cancel() }, 10000 );
}
else
{
alert( "Please request permissions first." );
}
Try it: Show Notification
Wrapping it Up
I hope someone found this useful. If you do anything cool with this library please let me know in the comments.
Warning: this is an experimental API that only works in Google Chrome right now. The API may change at any time and may be different when other browsers decide to implement similar functionality. Use with caution. Always check to make sure window.webkitNotifications !== undefined
.